AzCopy is a free command-line tool that is offered by Microsoft. It allows you to easily copy and transfer data (data migration) from and to Azure storage. It is designed for high performance transfers and can be deployed on both Windows (32-bit and 64-bit) and Linux systems, but also on macOS.
AzCopy for example allows users to copy data (blobs or files) between a file system and an Azure storage account, or between storage accounts. Users have the possibility to select items by specifying patterns, like wildcards or prefixes, to identify the needed files for upload or download. It currently supports Microsoft Azure Blob, File and Table storage.
If you’re interested, you can always find more information about AzCopy on this Microsoft Docs page
To automate the download and extraction process of this useful tool, I wrote the below PowerShell script which does all of the following:
- Check if the PowerShell window is running as Administrator (which is a requirement), otherwise the PowerShell script will be exited.
- Create the by user input specified management or tools folder (e.g. Wbin or AdminTools) on the C: drive if the folder not already exists.
- Delete the AzCopy folder and all files in the folder, if the folder already exists in the specified managment or tools folder.
- Download the latest AzCopy (Windows 64-bit) .zip file and save under the specified managment or tools folder.
- Extract the .zip file into the specified managment or tools folder folder.
- Delete the .zip file after extraction.
- Rename the azcopy_windows_*.* folder to AzCopy.
- Add the AzCopy folder, which holds the executable (.exe), to the PATH system variable for ease of use.
To use the script copy and save it as Download-and-Extract-AzCopy-to-specified-folder.ps1 or download it from GitHub. Then before using the script, adjust all variables to your use and then run the customized script with Administrator privileges from Windows Terminal, Visual Studio Code, or Windows PowerShell.
Prerequisites
- A workstation (Windows 10 or 11) or management server (Windows Server 2016, 2019 or 2022) with Internet connectivity.
PowerShell script
<#
.SYNOPSIS
A script used to download and extract the latest AzCopy Windows 64-bit executable (.exe) file into a specified foler (e.g. C:\Wbin\ folder).
.DESCRIPTION
The latest AzCopy Windows 64-bit executable (.exe) file will be downloaded from a static download link.
The downloaded .zip file will be extracted, and made availabele into the specified folder provided by the user when running the script.
At the end the directory location of the AzCopy executable will also be added to the system path for ease of use.
.NOTES
Filename: Download-and-Extract-AzCopy-to-specified-folder.ps1
Created: 02/03/21
Last modified: 10/01/22
Author: Wim Matthyssen
PowerShell: 5.1
Requires: -RunAsAdministrator
OS: Windows 10, Windows 11, Windows Server 2016, Windows Server 2019 and Windows Server 2022
Version: 2.0
Action: Change variables were needed to fit your needs.
Disclaimer: This script is provided "As Is" with no warranties.
.EXAMPLE
.\Download-and-Extract-AzCopy-to-specified-folder.ps1
.LINK
https://wmatthyssen.com/2021/03/03/powershell-azcopy-windows-64-bit-download-and-silent-installation/
#>
## ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
## Variables
$managementToolsFolderName = Read-Host "Please enter your management or administrator tools foldername (e.g. Wbin or AdminTools)" # Example: Wbin or AdminTools
$managementToolsFolderPath = "C:\" + $managementToolsFolderName +"\"
$itemType = "Directory"
$azCopyFolderName = "AzCopy"
$azCopyFolder = $managementToolsFolderPath + $azCopyFolderName
$azCopyUrl = "https://aka.ms/downloadazcopy-v10-windows"
$azCopyZip = $managementToolsFolderPath + "azcopy.zip"
$global:currenttime= Set-PSBreakpoint -Variable currenttime -Mode Read -Action {$global:currenttime= Get-Date -UFormat "%A %m/%d/%Y %R"}
$foregroundColor1 = "Red"
$foregroundColor2 = "Yellow"
$writeEmptyLine = "`n"
$writeSeperatorSpaces = " - "
## ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
## Check if PowerShell is running as Administrator, otherwise exit the script
$currentPrincipal = New-Object Security.Principal.WindowsPrincipal([Security.Principal.WindowsIdentity]::GetCurrent())
$isAdministrator = $currentPrincipal.IsInRole([Security.Principal.WindowsBuiltInRole]::Administrator)
if ($isAdministrator -eq $false) {
Write-Host ($writeEmptyLine + "# Please run PowerShell as Administrator" + $writeSeperatorSpaces + $currentTime)`
-foregroundcolor $foregroundColor1 $writeEmptyLine
exit
}
## ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
## Start script execution
Write-Host ($writeEmptyLine + "# Script started. Without any errors, it will need around 1 minute to complete" + $writeSeperatorSpaces + $currentTime)`
-foregroundcolor $foregroundColor1 $writeEmptyLine
## ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
## Create the specfied "management or tools folder" folder if not exists (e.g. Wbin or AdminTools)
If(!(test-path $managementToolsFolderPath))
{
New-Item -Path "C:\" -Name $managementToolsFolderName -ItemType $itemType -Force | Out-Null
}
Write-Host ($writeEmptyLine + "# $managementToolsFolderName folder available under the C: drive" + $writeSeperatorSpaces + $currentTime)`
-foregroundcolor $foregroundColor2 $writeEmptyLine
## ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
## Delete AzCopy folder if already exists in $adminToolsFolder folder
If(test-path $azCopyFolder)
{
Remove-Item $azCopyFolder -Recurse | Out-Null
}
Write-Host ($writeEmptyLine + "# $azCopyFolderName folder not available or existing one deleted" + $writeSeperatorSpaces + $currentTime)`
-foregroundcolor $foregroundColor2 $writeEmptyLine
## ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
## Download, extract and cleanup the latest AzCopy zip file
Invoke-WebRequest $azCopyUrl -OutFile $azCopyZip
Expand-Archive -LiteralPath $azCopyZip -DestinationPath $managementToolsFolderPath -Force
Remove-Item $azCopyZip
Write-Host ($writeEmptyLine + "# azcopy.exe available in extracted folder" + $writeSeperatorSpaces + $currentTime)`
-foregroundcolor $foregroundColor2 $writeEmptyLine
## ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
## Rename AzCopy version folder to AzCopy
$azCopyOriginalFolderName = Get-ChildItem -Path $managementToolsFolderPath -Name azcopy*
$azCopyFolderToRename = $managementToolsFolderPath + $azCopyOriginalFolderName
$azCopyFolderToRenameTo = $managementToolsFolderPath + $azCopyFolderName
Rename-Item $azCopyFolderToRename $azCopyFolderToRenameTo
Write-Host ($writeEmptyLine + "# $azCopyOriginalFolderName folder renamed to $azCopyFolderName" + $writeSeperatorSpaces + $currentTime)`
-foregroundcolor $foregroundColor2 $writeEmptyLine
## ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
## Add the AzCopy folder to the PATH System variable
[Environment]::SetEnvironmentVariable("Path", $env:Path + ";$azCopyFolderToRenameTo", "Machine")
Write-Host ($writeEmptyLine + "# The directory location of the AzCopy executable is added to the system path" + $writeSpace + $writeSeperatorSpaces + $currentTime)`
-foregroundcolor $foregroundColor2 $writeEmptyLine
## ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
## Write script completed
Write-Host ($writeEmptyLine + "# Script completed" + $writeSeperatorSpaces + $currentTime)`
-foregroundcolor $foregroundColor1 $writeEmptyLine
## ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
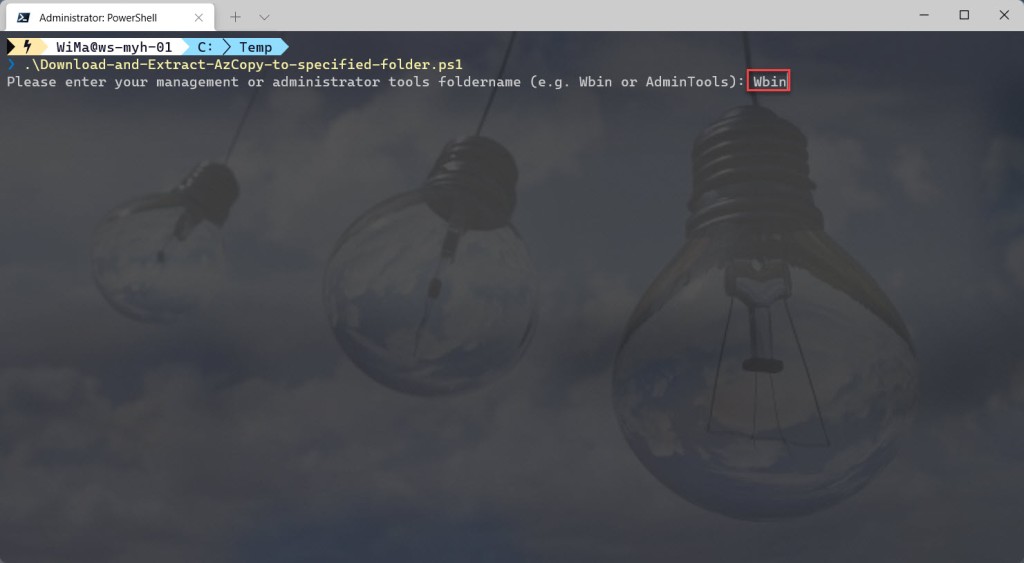
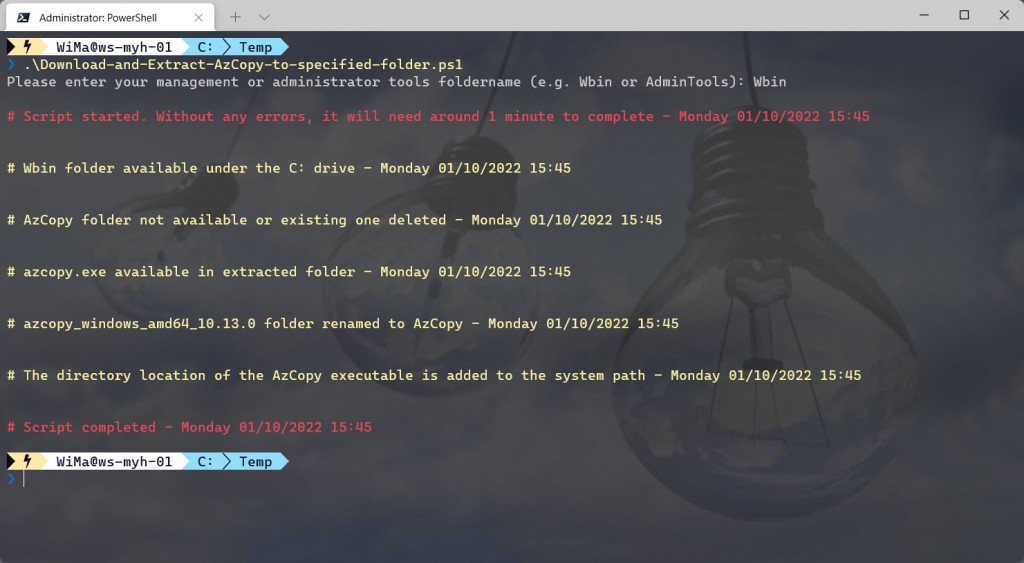
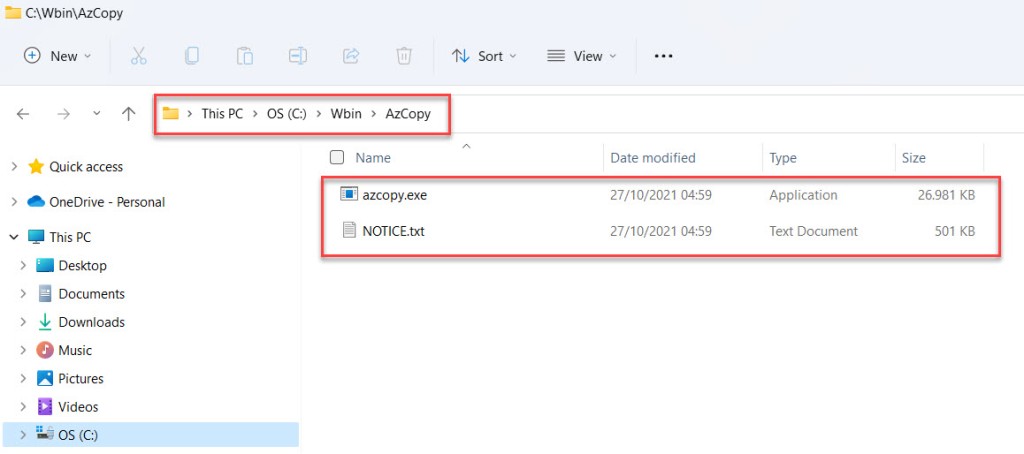
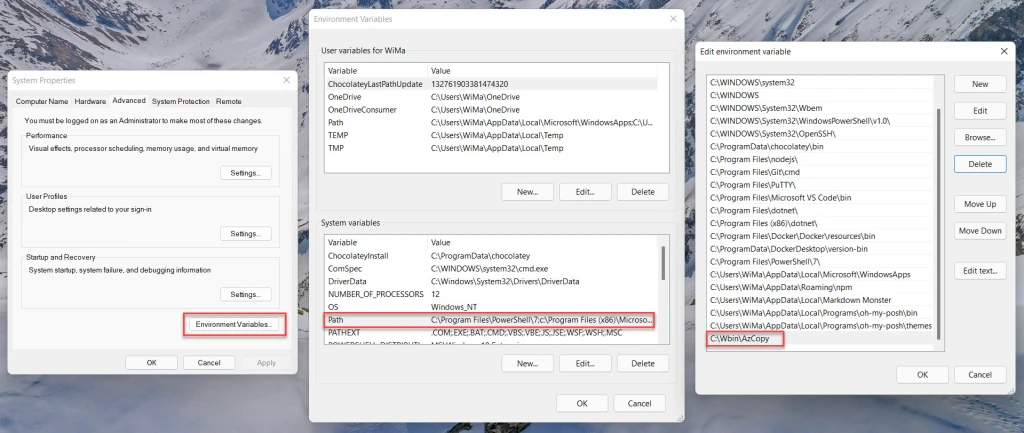
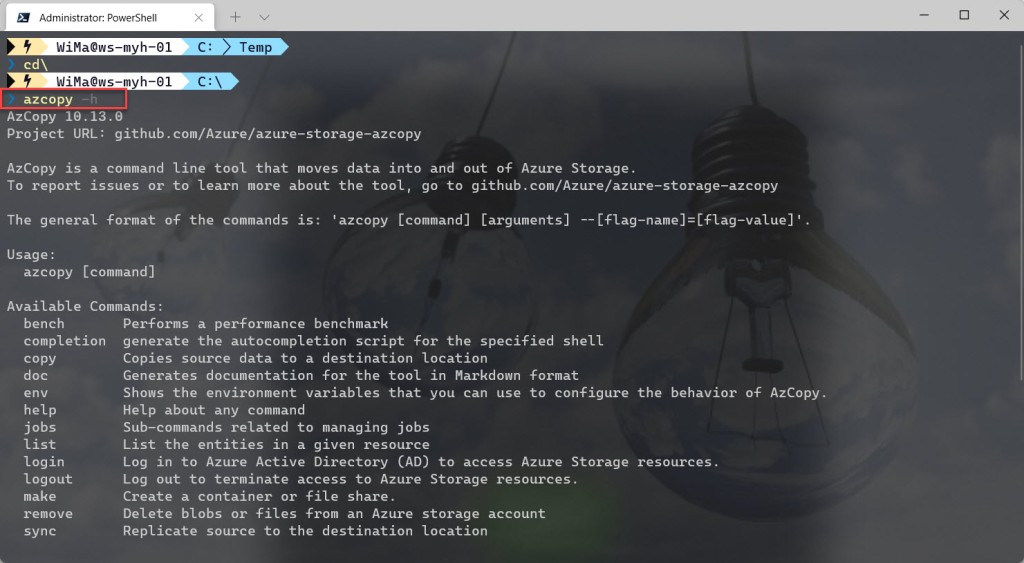
I hope this PowerShell script is useful for you and provides you with a good starting point to use AzCopy.
If you have any questions or recommendations about it, feel free to contact me through my Twitter handle (@wmatthyssen) or to just leave a comment.
Pretty nice script very useful, would you consider adding a block of script that checks for new versions of AzCopy given that MS releases newer versions very often ?
LikeLike
Hi Oscar, at the moment the script will deleted any previous versions of AzCopy, if the specified folder is the same as the previous one. And then the script always downloads and extracts the latest AzCopy version. I changed some commands so it now only takes less then a minute to run.
LikeLike
to avoid any folder delet that has azcopy in the prefix of the name. update line 110 to
$azCopyOriginalFolderName = Get-ChildItem -Path $managementToolsFolderPath -Name azcopy_*
LikeLike